Just recently, Facebook released the newest version of React, React 18. Being one of the most used JavaScript frameworks, we would of course like to address the latest release. After making some research I would like to give you a brief summary about what’s new and some examples of new hooks.
Speed is key
- The big selling point for React 18 is concurrency. This will affect the overall speed of the app and will help with updating state before rendering so no strange error will appear after weeks of testing locally. This is a process in the background that checks all the new state and decides when it is time to update the page.
- Automatic batching is getting better. Before React only batched updates inside React’s own event handlers but now it’s everywhere.
SSR is the new black
- There are lot of new improvements in the server-side rendering world that will make your life easier. But most is still in development. Facebook have however promised that server components will be an upcoming feature that will span the server and client.
- Suspense now works with SSR. This implies now that the UI will load and you don’t need to wait for anything else.
Good to know
- There are also some interesting changes in Strict mode. Now React will run more tests in development mode: mounts, simulate unmounting and mounting it again with the previous state.
- Facebook has been thinking of the upgrade process in this version. If you want to update your React project to version 18 you only need to configure one breaking change. React has updated the structure of the React-DOM and with that changed the import. Now you find the render methods createRoot and hydrateRoot for SSR in the React-DOM/client. See examples of previous method and new method:
import ReactDOM from 'react-dom';
import App from 'App';
const container = document.getElementById('app');
ReactDOM.render(<App />, container);
import ReactDOM from 'react-dom/client';
import App from 'App';
const container = document.getElementById('app');
// create a root
const root = ReactDOM.createRoot(container);
//render app to root
root.render(<App />);
Now to the fun part: The new hooks
Below are some new hooks that follow with React 18 and that we have looked into. Feel free to use the code snippets to your own projects.
useId
Is a hook that will create an unique id for both client and server. This will mainly help with library authors but I can’t see why we should add it in more places if we get issues with id:s.
useTransition
Is added so we as developers can decide which updates that are urgent and those we can put on hold. Example of how to use the useTransition hook:
import { useState, useTransition } from "react";
const SlowUI = ({ value }) => (
<>
{Array(value)
.fill(1)
.map((_, index) => (
<span key={index}>{value - index} </span>
))}
</>
);
function Transition() {
const [value, setValue] = useState(0);
const [value2, setValue2] = useState(100000);
const [isPending, startTransition] = useTransition();
const handleClick = () => {
//Will render directly
setValue(value + 1);
//Will rerender when loaded
startTransition(() => setValue2(value2 + 1));
};
return (
<>
<button onClick={handleClick}>{value}</button>
<div
style={{
opacity: isPending ? 0.5 : 1,
}}
>
<SlowUI value={value2} />
</div>
</>
);
}
export default Transition;
useDeferredValue
Is for defer re-rendering so the user can start clicking around the app until your slow api is back with some more data to render. Example of how to use the useTransition hook:
import { useDeferredValue, useState } from "react";
const SlowUI = () => (
<>
{Array(50000)
.fill(1)
.map((_, index) => (
<span key={index}>{100000} </span>
))}
</>
);
function DeferredValue() {
const [value, setValue] = useState(0);
const deferredValue = useDeferredValue(value);
const handleClick = () => {
setValue(value + 1);
};
return (
<>
{/* will rerender */}
<button onClick={handleClick}>{value}</button>
{/* will show old value until rerender */}
<div>DeferredValue: {deferredValue}</div>
<div>
<SlowUI />
</div>
</>
);
}
export default DeferredValue;
useSyncExternalStore
Is another hook for library authors. It allows external stores to support concurrent reads by forcing updates to be synchronous.
useInsertionEffect
Is a hook for css in javascript library’s to help performance of injecting styles in render
Summary
- There are some quality changes for the rendering that will make apps run smoother.
- There is a big focus on SSR which in turn gives us a hint that can tell us where Facebook thinks the web will move.
- The new hooks; some hooks for libraries that will make the platform more easy to use and build for.
This was all for now, subscribe for our blog to receive more news looking ahead.
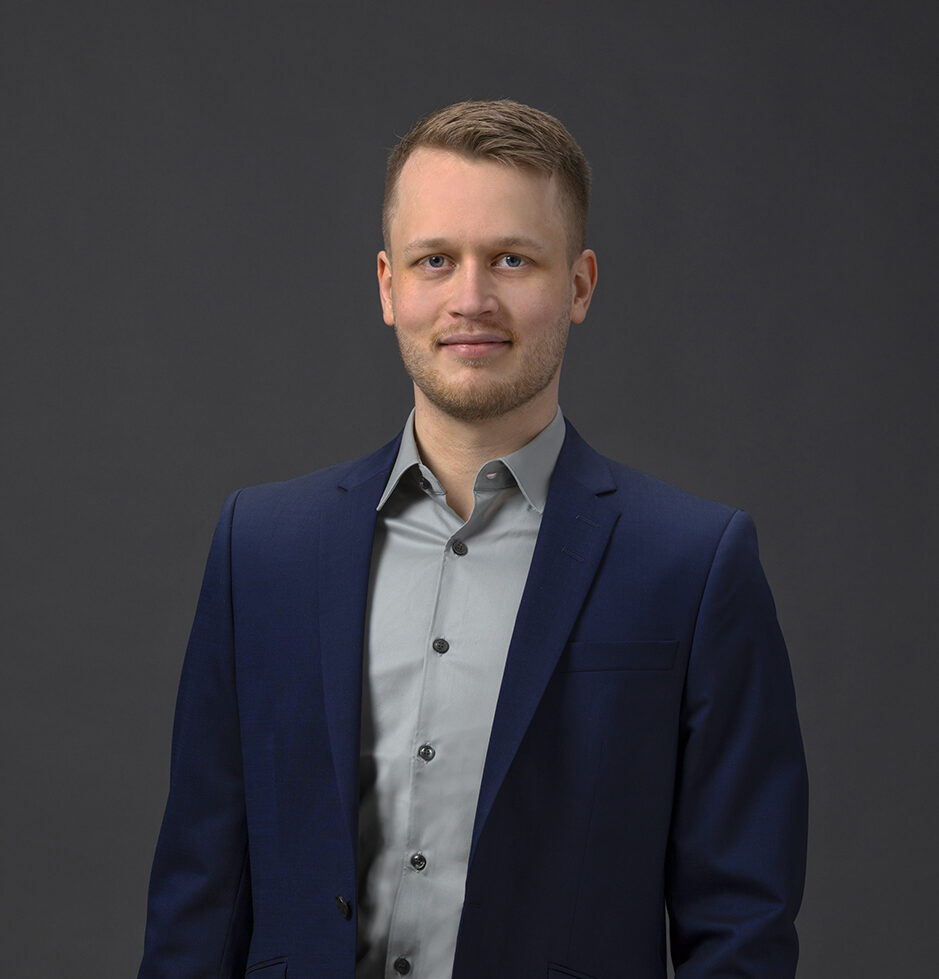
Author: Matthias Nyman
Fullstack developer that is interested in MMA and never say no to a spontaneous after work with friends.